terminal rendering
A unicode terminal renderer.
This project is perhaps my favourite dive into an unfamiliar topic. Getting to grips with rudimentary graphics programming has been foundational for my follow up projects. The project started with exploring Andy Sloane’s (a1k0n) ‘Donut math’ article, and has since led me to start learning OpenGL.
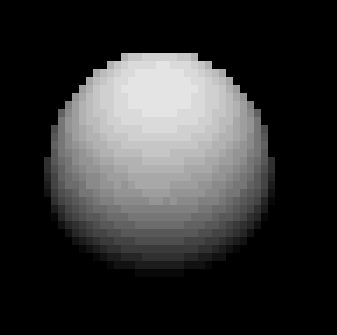
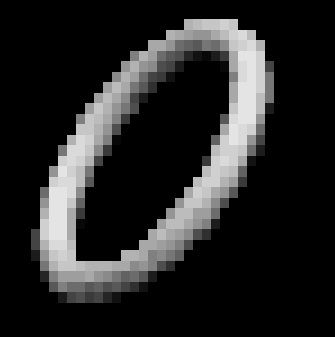
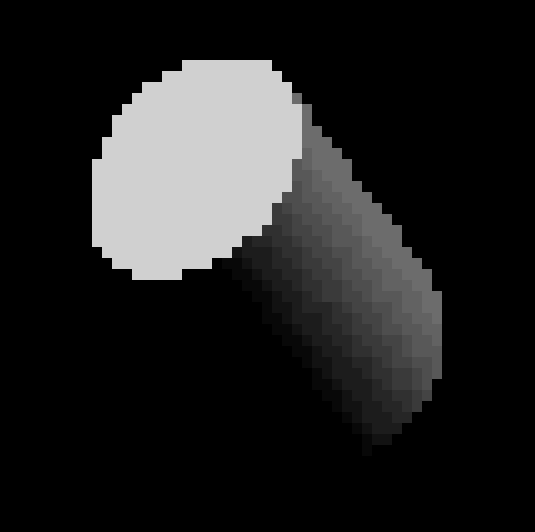
a1k0n’s approach uses the typical features you’d expect when rendering, Z-buffers and transforming 3D->2D using some nifty linear algebra, though employs a unique technique of iterating over the surface of the donut in three dimensions. I’ve retained this 3D iteration model to produce cubes, spheres, donuts, and a cylinder.
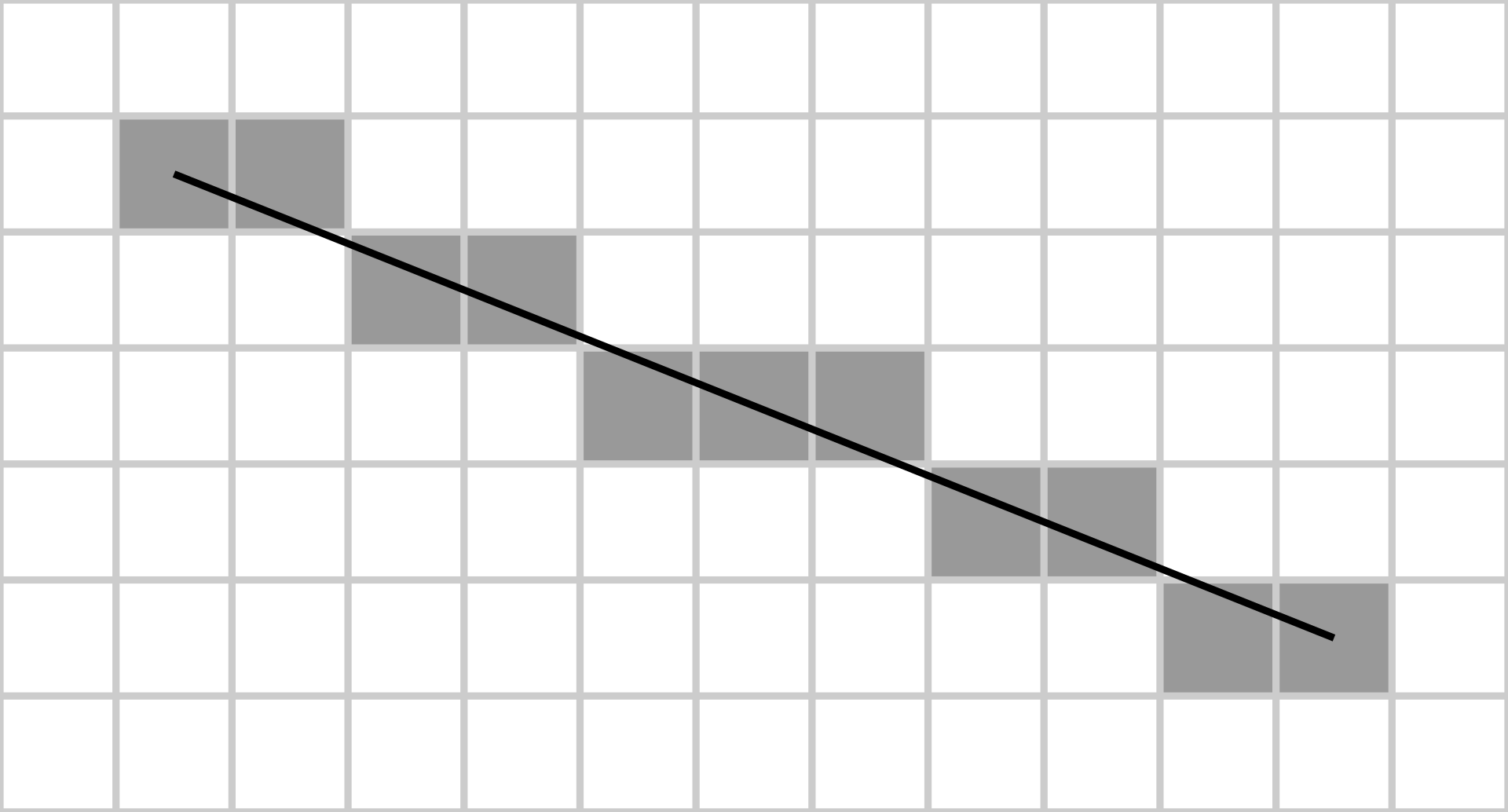
I’m considering replacing this with an implementation of Bresenham’s line algorithm and some form of triangle rasterisation, probably the scanline algorithm, for some nice rendering and a standard implementation of sub-pixel resolution.
#include <math.h>
#include <euch_vector.hxx>
#ifndef light_class
#define light_class
class light{
public:
euch_vector light_vec;
light();
light(float x_dir, float y_dir, float z_dir);
};
There’s also a few extra funky features, such as light no longer being hard-baked into objects and is an independant universal parallel source, like you’re on the moon. Probably the most distinct visual change is the utilisation of the unicode characters. This does cut down on the portability of the program, though the increase in rendering fidelity is more than worth it.
while (true){
dt = t_current - t_prev;
t_prev += dt;
t_acc += dt;
while( t_acc > t_rate ){
update();
t_acc -= t_rate;
}
draw()
}
My next update to this will probably involve moving to a (\Delta t) model of iteration with the proper accumulator and doing some calculations with respect to a previous or base state. This is really helpful as you can pin the execution of the physics to a specific rate, independent of the rendering process.
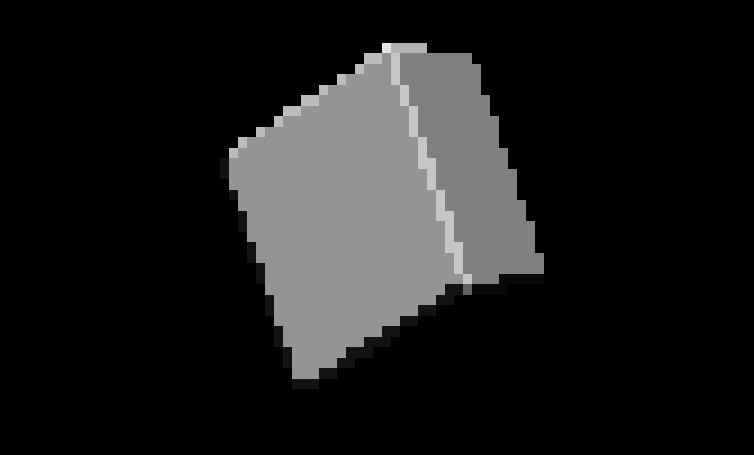
This will probably need some work in terms of the implemented Lorentz transforms in order to get the physics nice and correct. There’s also the question of what to do next with this. I wonder about it’s applications to my day to day work, and repeatedly land on if anyone needs to render ATLAS events quickly on the terminal?